6: Rotate the 8 ball while following the mouse
The next step is to move the white ball with the mouse to create the effect of the ball rotating.
I first set a mouse move event listener to trigger whenever the user moved their mouse.
$(window).on('mousemove', function(e){
// Calculate details for movement of white ball
});
Setting the travel constraints
Next was to set the minimum and maximum of X and Y so that the ball would not completely follow the mouse, but instead stay inside specified bounds.
I initially set the minimum and maximum of X and Y to be the width of the magic 8 ball's dark base:
z-index: 10;
width: 509px;
height: 509px;
max-height: 60vh;
max-width: 60vh;
overflow: hidden;
border-radius: 50%;
However, this changed later as it ended up resulting in the white ball going too far off the side (as shown below).
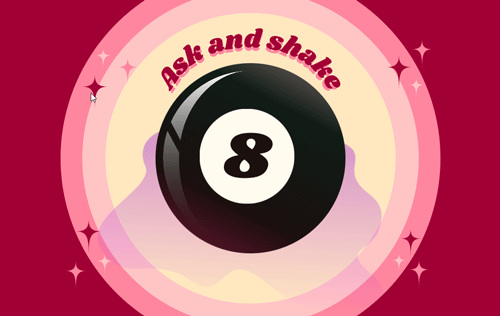
Due to this, I decided to create an additional div around the white ball that had a smaller width and height to limit the movement of the white ball so it would not go too far off the edge of the dark base.
z-index: 10;
width: 409px;
height: 409px;
max-height: 40vh;
max-width: 40vh;
border-radius: 50%;
Now that this was set, the next important thing to do is to set the white ball's position to be relative so that it moves relative to its initial position, rather than the page position.
#image8BallWhiteCircle {
z-index: 4;
max-height: 30vh;
position: relative;
}
Now to set the minimum and maximum of the X and Y position on the mouse move event.
The first section was to set the minimum and maximum of the now smaller div wrapping the white ball to specify how far the white ball can travel.
$(window).on('mousemove', function(e){
minX = 0;
maxX = minX + $('#divSmallerMoveAreaWhiteCircle').width();
minY = 0;
maxY = minY + $('#divSmallerMoveAreaWhiteCircle').height();
});
Creating the x and y event positions
Still within the mousemove event, the next step was to calculate the e.pageX and e.pageY event to get the coordinates of the mouse, and then to create a variable to store the x and y position of the white ball, while keeping in the bounds of the minimum and maximum of the wrapping div.
var x = Math.max(
Math.min(
e.pageX, maxX
), minX);
var y = Math.max(
Math.min(
e.pageY, maxY
), minY);
Now that has been set, still within the mousemove event, the final step is to apply this to the white ball.
$('#image8BallWhiteCircle').css('left', x + 'px');
$('#image8BallWhiteCircle').css('top', y + 'px');
This then results in the below in a more limited movement of the white ball within the magic 8 ball:
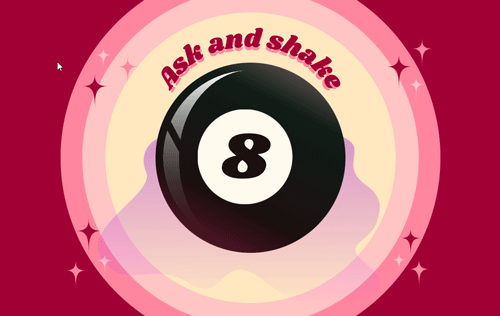