12: Creating and animating bubbles
Now there is one more thing I wanted to include to add to that nostalgia of the Magic 8 Ball that you would shake vigorously as a kid.
Whenever I shook anyones 8 Ball, I always noticed a bunch of bubbles, usually covering the text and going in random directions.
Creating the bubble image
So to add bubbles into this 8 ball, I first created a single bubble in Figma and exported it as an SVG as I would be resizing it once inside the site.
I then added the bubble 3 times into the page, again centering them with the CSS as the other images (see Tutorial 2).
Setting the scale
For these bubbles, I then set slightly different sizes for each added so that there was some variation to the bubbles.
#image8BallBubble1{
z-index: 7;
max-height: 3vh;
position: relative;
}
#image8BallBubble2 {
z-index: 7;
max-height: 2.5vh;
position: relative;
}
#image8BallBubble3 {
z-index: 7;
max-height: 2vh;
position: relative;
}
Randomising the position
I then created a function that set a minimum and maximum x and y constraints that the bubbles could travel within, and got a random x and y coordinate within this range.
function calcBubbleCoordinates(){
bMinX = 0;
bMaxX = bMinX + $('#divWrapimage8BallResult').width();
bMinY = 0;
bMaxY = bMinY + $('#divWrapimage8BallResult').height();
var x = getRandomInt(bMinX, bMaxX);
var y = getRandomInt(bMinY, bMaxY);
return {x, y};
}
Creating the bubble travel
Then within the fadeInResult() function, I got a random location for the each bubble to travel to when the results started to fade in.
var bubble1XY = calcBubbleCoordinates();
var bubble2XY = calcBubbleCoordinates();
var bubble3XY = calcBubbleCoordinates();
$('#image8BallBubble1').css('left', bubble1XY.x + 'px');
$('#image8BallBubble1').css('top', bubble1XY.y + 'px');
$('#image8BallBubble2').css('left', bubble2XY.x + 'px');
$('#image8BallBubble2').css('top', bubble2XY.y + 'px');
$('#image8BallBubble3').css('left', bubble3XY.x + 'px');
$('#image8BallBubble3').css('top', bubble3XY.y + 'px');
I then created a fade in CSS animation, the purpose being to add each bubble when the result faded in.
.bubble1FadeIn {
animation: bubble1FadeIn 3s;
animation-iteration-count: infinite;
transition-timing-function: ease-in-out;
}
@keyframes bubble1FadeIn {
0% { opacity: 0; transform: translate(0px, 0px)}
100% { opacity: 1; transform: translate(30px, 50px)}
}
Within the fadeInResult() function, I first added the bubble fade in class to each so they would start to fade in.
I then set a css transform: translate to 3 different directions for set pixels so that the bubbles would move in different directions from their initial random location. Then once the animation completed, I set the opacity to 1 so that they would stay visible and removed the bubble fade in class.
$('#image8BallBubble1').addClass('bubble1FadeIn');
$('#image8BallBubble2').addClass('bubble2FadeIn');
$('#image8BallBubble3').addClass('bubble3FadeIn');
setTimeout(function(){
$('#image8BallBlueTriangle, #htmlResultText').css('opacity', '1');
$('#image8BallBlueTriangle, #htmlResultText').removeClass('fadeInTri');
}, 2500);
setTimeout(function(){
$('#image8BallBubble1').css('transform', 'translate(30px, 50px)');
$('#image8BallBubble2').css('transform', 'translate(-30px, -25px)');
$('#image8BallBubble3').css('transform', 'translate(-20px, 40px)');
$('#image8BallBubble1, #image8BallBubble2, #image8BallBubble3').css('opacity', '1');
$('#image8BallBubble1').removeClass('bubble1FadeIn');
$('#image8BallBubble2').removeClass('bubble2FadeIn');
$('#image8BallBubble3').removeClass('bubble3FadeIn');
}, 3000);
Finally for the fade out result, I added the existing fadeOutTri class to the bubbles to fade out at the same time.
This then resulted in the below:
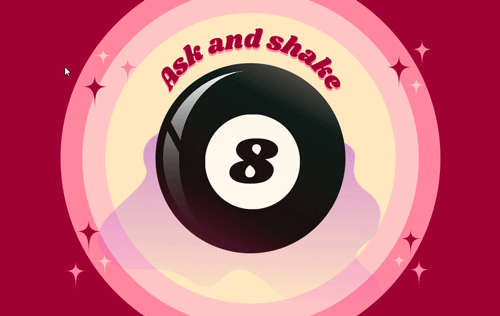