10: Choosing and displaying the result text
Next is to choose the result text and animate it.
Creating the potential outcomes
In order to do this however, we need to know firstly what are the possible results and how we are going to choose the result.
According to Wikipedia,
A standard Magic 8 Ball has twenty possible answers, including ten affirmative answers (●), five non-committal answers (●), and five negative answers (●).
The above Wikipedia article also listed all possible answers in a standard Magic 8 ball.
From this, I created an array of all the possible answers, and then created a function to randomly choose a result and return it in all uppercase letters.
function getRandomResult() {
var resultArr = ["It is certain", "It is decidedly so", "Without a doubt", "Yes definitely", "You may rely on it", "As I see it, yes", "Most likely", "Outlook good", "Yes", "Signs point to yes", "Reply hazy, try again", "Ask again later", "Better not tell you now", "Cannot predict now", "Concentrate and ask again", "Don't count on it", "My reply is no", "My sources say no", "My sources say no", "Very doubtful"];
var arrInt = Math.floor((Math.random() * 19));
return resultArr[arrInt].toUpperCase();
}
Creating the result fade animation
Once the result had been chosen, I then created CSS to animate the opacity change, and another function to fade the result in and set the HTML to the chosen result via the returned value in the getRandomResult() function.
.fadeInTri {
animation: fadeInTriangle 2.5s;
animation-iteration-count: infinite;
transition-timing-function: ease-in-out;
}
function fadeInResult(){
$('#htmlResultText').html(getRandomResult());
$('#image8BallBlueTriangle, #htmlResultText').addClass('fadeInTri');
setTimeout(function(){
$('#image8BallBlueTriangle, #htmlResultText').css('opacity', '1');
$('#image8BallBlueTriangle, #htmlResultText').removeClass('fadeInTri');
}, 2500);
}
Communicating clickable events to the user
Additionally, to let the user know that the 8 ball is clickable, I set the cursor to be a pointer when hovering over the ball.
To stop additional clicks from interrupting the animation, after clicking the ball and during the animation, I changed the cursor to stars and blocked any clicks until the animation had completed.
Once the animation was complete, I then changed the cursor back to a pointer so that the user was able to see that they were now able to click to shake the 8 ball again.
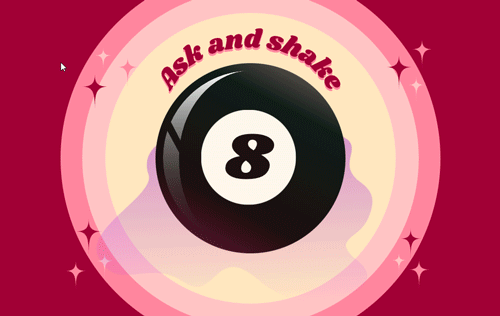